To say that Rust is the programming language of the future, as many in the industry have claimed, is not an overstatement. Rust has been quickly growing in popularity and demand since its official public launch in 2015, and computer programming is a highly desired skill in the job market today. If you’ve never taken a crack at it, this might be a great opportunity for you to try it out: Google is launching a new free online course for people to learn how to use Rust, a programming language designed to be safe, concurrent, and efficient.
Rust offers a unique combination of performance and safety, making it an excellent choice for a wide range of applications, from system programming to web development. The language’s ownership model ensures memory safety without needing a garbage collector, which can often lead to performance overhead in other languages.
In this guide, we will dive deep into the details of the comprehensive online course designed to teach Rust to beginners who have experience in coding, mainly in languages like C++ or Java. This course is structured to take approximately four days to complete, covering Rust syntax and language fundamentals through modifying existing programs and writing new ones.
Course Overview
The course is meticulously designed for beginners who have experience in coding but are new to Rust. It spans four days, with each day focusing on different aspects of the Rust language. The course covers everything from basic syntax to advanced concepts like concurrency and interoperability. By the end of the course, learners will have a solid foundation in Rust and be able to write efficient, safe, and concurrent programs.
To access the course, simply follow this link to GitHub to start the course. There is no need to register or login to begin.
Day 1: Basics and Fundamentals
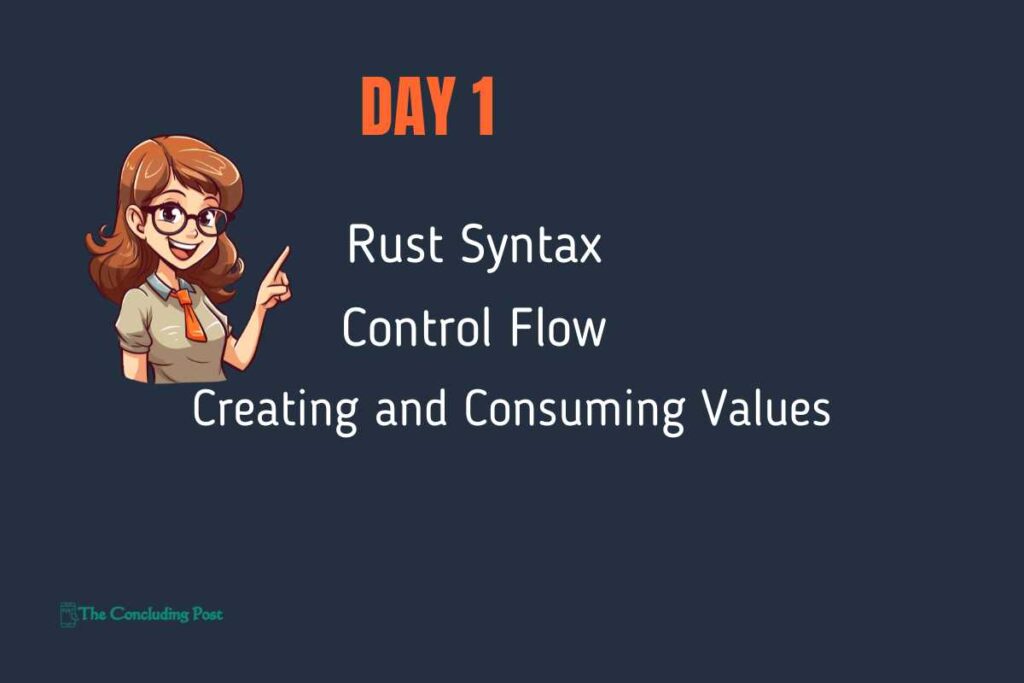
Rust Syntax
- Objective: Understand the basic syntax and structure of Rust.
- Content: The first module introduces learners to the basic syntax of Rust, covering variables, data types, functions, and control flow constructs like if-else statements and loops. The goal is to get learners comfortable with the structure and syntax of Rust programs.
- Activities: Simple exercises to get familiar with writing and running Rust programs. These exercises will involve writing basic Rust programs that demonstrate the use of variables, control flow, and functions.
Control Flow
- Objective: Learn how to use loops, if-else statements, and match statements in Rust.
- Content: This section dives deeper into control flow mechanisms in Rust, explaining how to use loops (for, while, and loop), if-else statements, and match statements to control the flow of a program. Examples will illustrate the usage and best practices for these constructs.
- Activities: Practice problems to reinforce understanding of control flow constructs. Learners will write programs that use various control flow statements to solve common programming problems.
Creating and Consuming Values
Objective: Get introduced to variables, constants, and the concept of immutability.
Content: This module explains how Rust handles variable binding, mutability, and shadowing. Learners will understand the difference between mutable and immutable variables and how to use constants in Rust.
Activities: Coding exercises to practice creating and using variables and constants. Learners will write programs that demonstrate the use of mutable and immutable variables and constants, and understand how Rust enforces these rules to ensure safety.
Day 2: Memory Management and Ownership
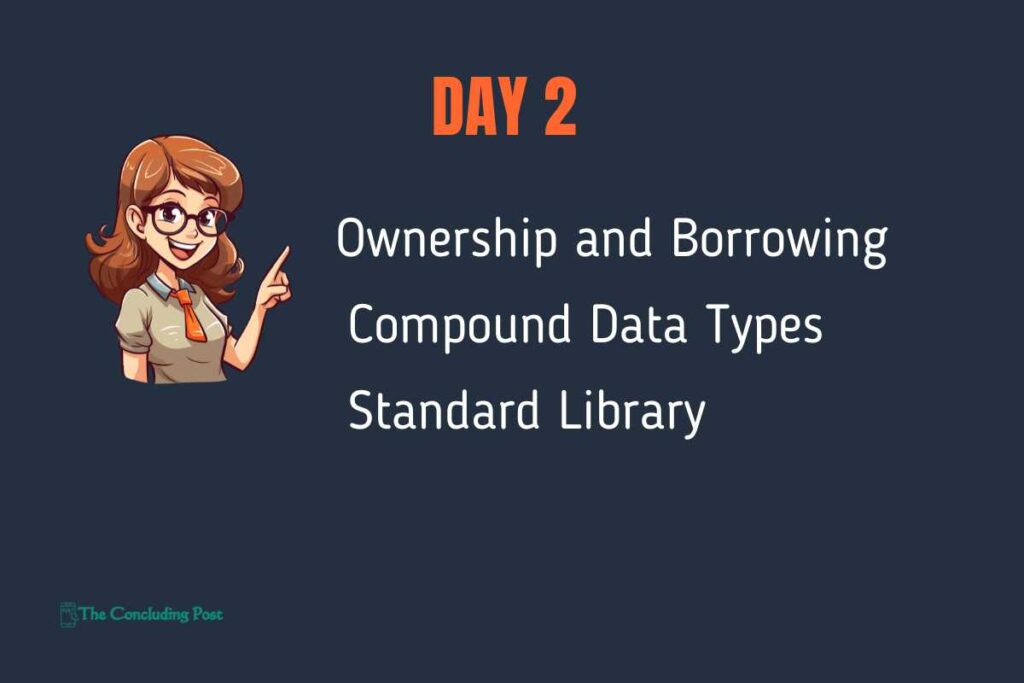
Ownership and Borrowing
Objective: Understand Rust’s unique ownership system, which ensures memory safety.
Content: One of Rust’s standout features is its ownership system. This section provides an in-depth explanation of ownership, borrowing, and lifetimes in Rust. Learners will understand how Rust manages memory and prevents common errors like null pointer dereferencing and data races.
Activities: Hands-on examples and exercises to illustrate ownership and borrowing. Learners will write programs that demonstrate how Rust’s ownership model works in practice, including examples of borrowing and lifetimes.
Compound Data Types
Objective: Explore structs, enums, and tuples.
Content: Rust provides several ways to define compound data types. This module covers structs, enums, and tuples, explaining how to define and use them. Learners will understand when and how to use each of these types to model complex data.
Activities: Practical exercises to create and manipulate compound data types. Learners will write programs that use structs, enums, and tuples to solve real-world problems.
Standard Library
Objective: Utilize Rust’s standard library to manage collections and perform common tasks.
Content: This section provides an overview of Rust’s standard library, focusing on collections like Vec and HashMap. Learners will understand how to use the standard library to manage collections and perform common tasks efficiently.
Activities: Coding exercises to practice using the standard library. Learners will write programs that use Vec, HashMap, and other standard library features to solve practical problems.
Day 3: Advanced Concepts
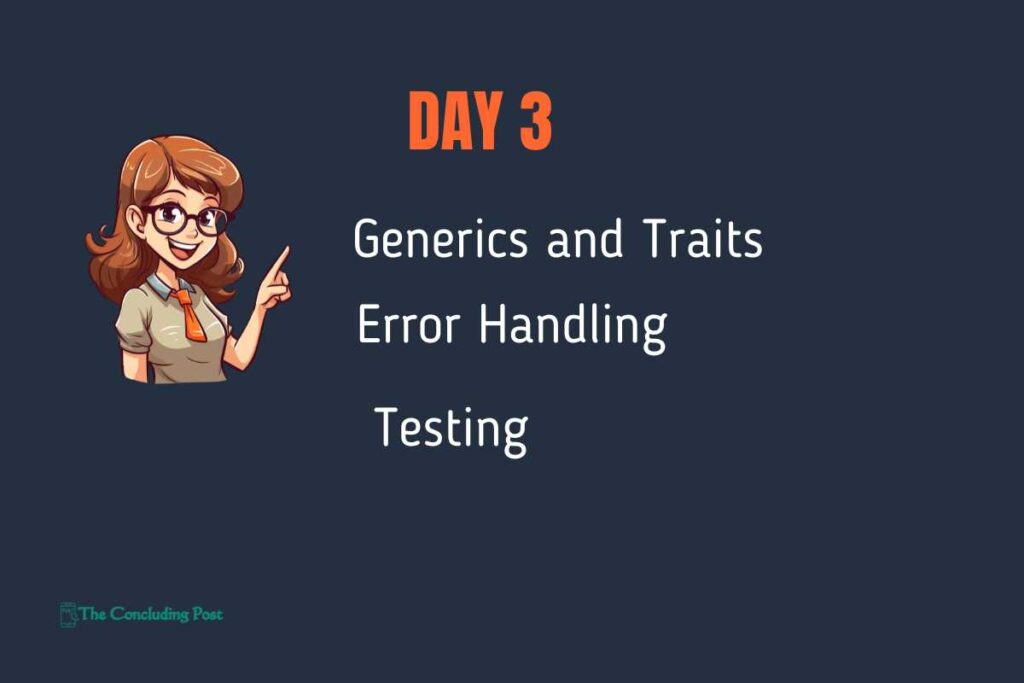
Generics and Traits
Objective: Learn how to write flexible and reusable code using generics and traits.
Content: Generics and traits are powerful features in Rust that enable code reuse and abstraction. This module introduces generics and traits, explaining how to define and use them. Learners will understand how to write flexible and reusable code using these features.
Activities: Practice problems to implement generics and traits in Rust programs. Learners will write programs that use generics and traits to solve problems in a generic and abstract way.
Error Handling
Objective: Implement robust error handling using Result and Option types.
Content: Rust provides powerful mechanisms for error handling using the Result and Option types. This section explains how to use these types to handle errors and optional values safely and idiomatically.
Activities: Coding exercises to practice error handling in Rust. Learners will write programs that demonstrate how to use Result and Option types to handle errors and optional values.
Testing
Objective: Write tests to ensure code correctness and reliability.
Content: Testing is an essential part of software development. This module introduces Rust’s testing framework and best practices for writing tests. Learners will understand how to write and run tests to ensure code correctness and reliability.
Activities: Writing and running tests for Rust programs. Learners will write tests for their Rust programs and run them to ensure their code works as expected.
Day 4: Practical Applications
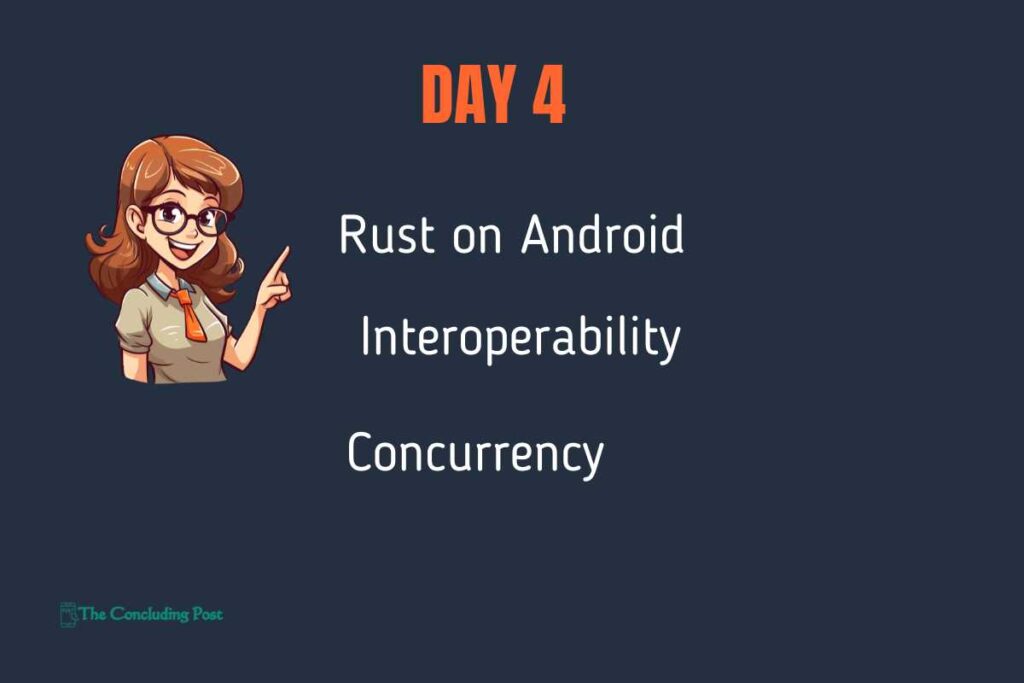
Rust on Android
Objective: Get an introduction to using Rust for Android platform development.
Content: Rust can be used to develop applications for the Android platform. This section provides an overview of setting up a Rust development environment for Android and building basic applications.
Activities: Simple project to create an Android application using Rust. Learners will set up their development environment and build a basic Android application using Rust.
Interoperability
Objective: Learn how Rust interacts with C, C++, and Java.
Content: Rust’s interoperability features allow it to call functions from other languages like C, C++, and Java. This module explains how to use Rust’s FFI (Foreign Function Interface) to interact with code written in other languages.
Activities: Practical exercises to implement interoperability in Rust programs. Learners will write Rust programs that call functions from C, C++, and Java, demonstrating Rust’s interoperability features.
Concurrency
Objective: Explore Rust’s approach to concurrency, including threads and async/await.
Content: Rust provides powerful features for concurrent programming, including threads and asynchronous programming using async/await. This section explains Rust’s concurrency model and how to use these features to write concurrent programs.
Activities: Coding exercises to implement concurrent and asynchronous programs in Rust. Learners will write programs that use threads and async/await to solve concurrent programming problems.
Rust Learning Program
Day | Module | Objective | Content | Activities |
---|---|---|---|---|
Day 1 | Rust Syntax | Understand the basic syntax and structure of Rust. | Introduction to Rust syntax: variables, data types, functions, control flow constructs (if-else statements, loops). | Simple exercises to write and run basic Rust programs. |
Control Flow | Learn to use loops, if-else statements, and match statements in Rust. | Detailed explanation of control flow mechanisms in Rust: loops (for, while, loop), if-else statements, match statements. | Practice problems to reinforce control flow constructs by writing programs using these statements. | |
Creating and Consuming Values | Introduce variables, constants, and immutability. | Explanation of variable binding, mutability, and shadowing in Rust. Differences between mutable and immutable variables, and how to use constants. | Coding exercises to create and use variables and constants, demonstrating Rust’s enforcement of safety. | |
Day 2 | Ownership and Borrowing | Understand Rust’s ownership system for memory safety. | In-depth explanation of ownership, borrowing, and lifetimes in Rust. Understanding how Rust manages memory and prevents errors like null pointer dereferencing and data races. | Hands-on examples and exercises to illustrate ownership and borrowing by writing programs demonstrating Rust’s ownership model. |
Compound Data Types | Explore structs, enums, and tuples. | Explanation of defining and using compound data types: structs, enums, tuples. Understanding when and how to use each type to model complex data. | Practical exercises to create and manipulate compound data types by writing programs using structs, enums, and tuples. | |
Standard Library | Utilize Rust’s standard library for collections and common tasks. | Overview of Rust’s standard library, focusing on collections like Vec and HashMap. Understanding how to manage collections and perform common tasks. | Coding exercises to practice using the standard library by writing programs using Vec, HashMap, and other standard library features. | |
Day 3 | Generics and Traits | Write flexible and reusable code using generics and traits. | Introduction to generics and traits, how to define and use them. Understanding how to write flexible and reusable code using these features. | Practice problems to implement generics and traits in Rust programs by writing programs using these features. |
Error Handling | Implement robust error handling using Result and Option types. | Explanation of using Result and Option types for error handling and optional values safely and idiomatically. | Coding exercises to practice error handling by writing programs using Result and Option types. | |
Testing | Write tests to ensure code correctness and reliability. | Introduction to Rust’s testing framework and best practices for writing tests. Understanding how to write and run tests to ensure code correctness. | Writing and running tests for Rust programs by writing tests and running them to ensure code works as expected. | |
Day 4 | Rust on Android | Introduction to using Rust for Android development. | Overview of setting up a Rust development environment for Android and building basic applications. | Simple project to create an Android application using Rust by setting up the development environment and building a basic app. |
Interoperability | Learn how Rust interacts with C, C++, and Java. | Explanation of Rust’s FFI (Foreign Function Interface) to interact with code written in C, C++, and Java. | Practical exercises to implement interoperability in Rust programs by writing Rust programs calling functions from other languages. | |
Concurrency | Explore Rust’s approach to concurrency, including threads and async/await. | Explanation of Rust’s concurrency model, including threads and asynchronous programming using async/await. | Coding exercises to implement concurrent and asynchronous programs in Rust by writing programs using threads and async/await. |
Why Rust?
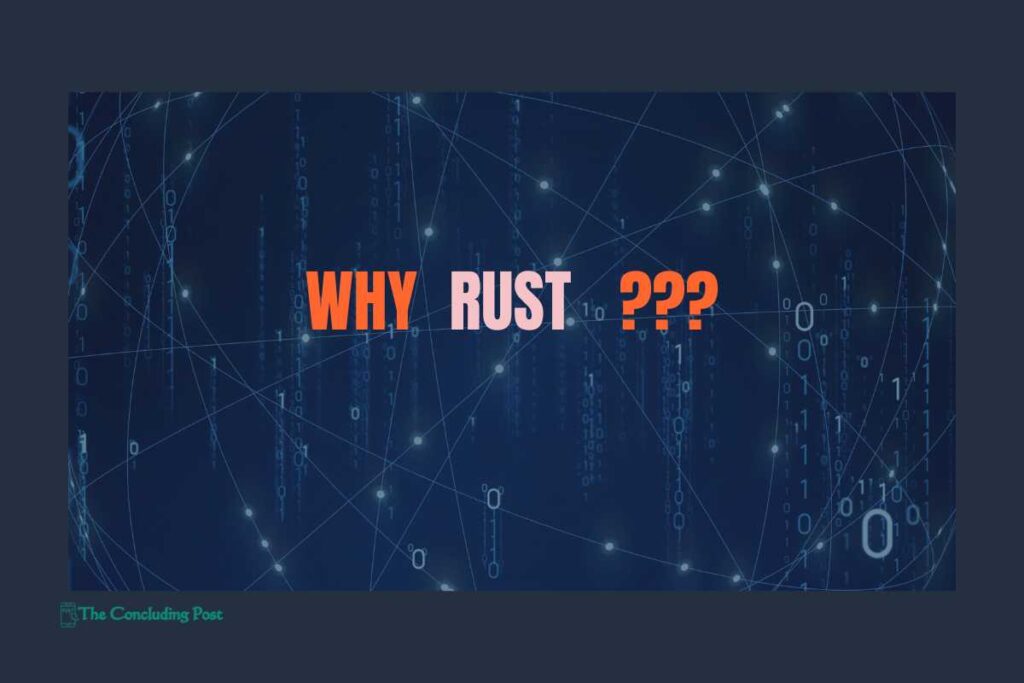
Back when we didn’t have the printing press, most writing was done by hand. This required a lot more effort and time to produce written products, and it was easier to make errors. Although writing by hand gave people more control, it also exposed their work to mistakes. A single error could undermine the credibility of the work and could mean starting over.
The emergence of Rust in the coding world is similar to the emergence of the printing press. You can think of writing by hand as C and C++, and Rust as the printing press. A lot of our older systems are written in C and C++, meaning it’s not unusual to find bugs or errors in the code. Once Rust came onto the scene, many companies started adopting the language and even transcribing a lot of their previous C and C++ work into Rust to make them less likely to have bugs, safer from hackers, and faster by taking less memory and processing power—about half as much electricity, according to a recent study.
Rust’s safety features, such as its ownership system, ensure memory safety without the need for a garbage collector, which can introduce performance overhead in other languages. This makes Rust an ideal choice for system programming, where performance and safety are critical. Additionally, Rust’s concurrency model allows developers to write safe and efficient concurrent programs, addressing the needs of modern applications that require high performance and scalability.
Job Opportunities
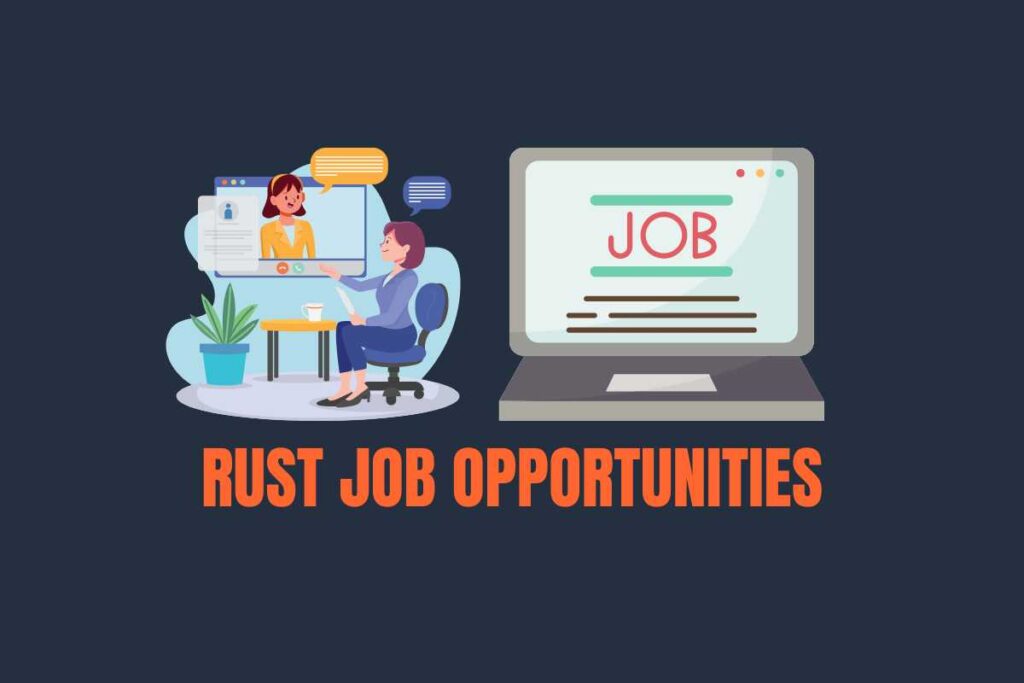
Having experience working with Rust is something many employers are looking for. RustJobs is a whole job board dedicated to employers searching for people who know how to use it. Some jobs, like this remote job from Jobot, require you to design, build, and maintain blockchain infrastructure using Rust—with a starting salary of $150,000 to $500,000.
Rust’s growing popularity means that the demand for Rust developers is on the rise. Companies across various industries, including tech giants like Amazon, Google, Meta, and Microsoft, are adopting Rust for their critical projects. This has created a robust job market for Rust developers, with competitive salaries and opportunities to work on cutting-edge technologies.
In addition to blockchain, Rust is being used in various domains such as web development, system programming, game development, and embedded systems. This versatility makes Rust an attractive skill for developers looking to
diversify their expertise and explore different career paths. Here are some areas where Rust expertise is particularly valuable:
- System Programming:
Rust’s safety and performance features make it an ideal choice for system programming. Companies developing operating systems, device drivers, and other low-level software components are increasingly looking for Rust developers. Notable examples include the Redox OS project, which is an entire operating system written in Rust, and companies like Mozilla, which uses Rust in the development of their browser engine, Servo.
2. Web Development:
Rust is making significant inroads into web development with frameworks like Rocket and Actix. These frameworks leverage Rust’s performance and safety to build high-performance, secure web applications. Companies looking to build scalable web services and APIs are starting to adopt Rust for its reliability and efficiency.
3. Game Development:
The game development industry is always on the lookout for languages that can deliver high performance. Rust, with its zero-cost abstractions and efficient memory management, is becoming a popular choice for game developers. Libraries like Amethyst and Bevy are bringing Rust into the realm of game development, allowing developers to create fast and safe games.
4. Blockchain and Cryptography:
Blockchain technology requires high levels of security and performance. Rust’s memory safety and concurrency model make it an excellent choice for developing blockchain applications. Many blockchain projects, including Polkadot and Solana, are built using Rust. Rust’s capabilities in cryptography also make it a preferred language for developing secure cryptographic algorithms and protocols.
5. Embedded Systems:
Rust’s performance and safety features are also valuable in the domain of embedded systems, where resource constraints and the need for reliability are paramount. The language’s ability to produce efficient, low-level code makes it suitable for writing firmware and other software for embedded devices.
Learning Outcomes
By the end of this course, learners will have gained:
- Comprehensive Understanding of Rust Syntax: Learners will be able to write and understand Rust programs using variables, functions, control flow constructs, and basic data structures.
- Deep Knowledge of Ownership and Memory Safety: Learners will understand Rust’s unique ownership system and how it ensures memory safety. They will be able to apply these concepts to write safe and efficient Rust programs.
- Proficiency in Using Compound Data Types: Learners will be adept at using structs, enums, and tuples to model complex data in Rust. They will also be familiar with Rust’s standard library and how to use it effectively.
- Ability to Implement Generics and Traits: Learners will be able to write flexible and reusable Rust code using generics and traits. They will understand how to leverage these features to create abstract and generic solutions.
- Robust Error Handling Skills : Learners will know how to handle errors and optional values in Rust using the Result and Option types. They will be able to write robust and reliable Rust programs that handle errors gracefully.
- Testing and Debugging Proficiency: Learners will be familiar with Rust’s testing framework and best practices for writing tests. They will be able to write and run tests to ensure their code is correct and reliable.
- Experience with Practical Applications: Learners will gain hands-on experience with practical applications of Rust, including developing for the Android platform, interoperability with other languages, and writing concurrent and asynchronous programs.
Conclusion
This course offers a thorough introduction to Rust, covering everything from the basics to advanced topics like concurrency and interoperability. With Rust’s growing popularity and demand, learning this language opens up numerous opportunities for developers in various domains. Whether you’re interested in system programming, web development, game development, blockchain, or embedded systems, Rust provides the tools and features to build safe, efficient, and high-performance software.
Embarking on this journey with Rust not only enhances your programming skills but also positions you at the forefront of modern software development practices. The course is designed to be comprehensive yet accessible, ensuring that by the end of it, you will have the confidence and knowledge to tackle real-world problems using Rust.